Backtesting Crypto Trading Strategies with Python C++ 2025
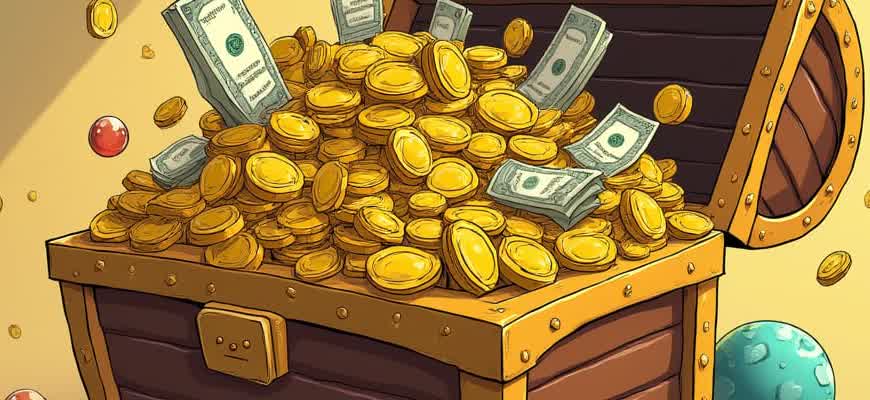
With the growing complexity of the cryptocurrency markets and the rapid evolution of trading strategies, backtesting has become an essential process for traders and developers. By using backtesting, strategies can be evaluated under historical market conditions to assess their performance and minimize potential risks. In this context, Python and C++ have emerged as two of the most popular programming languages for implementing these backtests, offering both high-level ease of use and low-level computational efficiency.
Python, with its rich ecosystem of libraries such as Pandas, NumPy, and TA-Lib, provides an accessible framework for designing and testing algorithmic strategies. On the other hand, C++ offers a significant performance advantage, particularly for executing high-frequency strategies that require rapid decision-making. The combination of these two languages allows traders to optimize their algorithms for both ease of development and execution speed.
- Python: Ideal for rapid development and data manipulation.
- C++: Preferred for high-performance, latency-sensitive applications.
- Integration: Many traders combine Python for strategy development and C++ for final execution.
Important: Backtesting results may not always accurately reflect real market conditions due to factors like slippage, transaction costs, and market impact.
To effectively backtest crypto trading strategies, developers often rely on data structures that can handle large datasets, real-time updates, and complex calculations. A well-optimized backtest is key to ensuring that a strategy is robust enough for live trading.
Factor | Python | C++ |
---|---|---|
Development Speed | High | Low |
Execution Speed | Moderate | High |
Libraries & Tools | Pandas, NumPy, TA-Lib | Boost, Eigen |
Setting Up a Python Environment for Crypto Backtesting
To start building a backtesting environment for crypto trading, you need a well-structured Python setup that supports high-performance calculations and data handling. Crypto market data is often complex, with high frequency and large volumes, requiring efficient processing and robust libraries. The first step in setting up your environment is installing the necessary packages and tools. These packages will help you collect, analyze, and test your strategies efficiently.
Python provides a rich ecosystem of libraries specifically tailored for financial modeling and backtesting. These libraries handle everything from data extraction to strategy implementation and performance evaluation. Below is a breakdown of the key components you'll need to set up your Python environment for backtesting crypto trading strategies.
Required Python Libraries
- Pandas: Essential for handling time-series data and performing data analysis tasks like data cleaning, manipulation, and aggregation.
- NumPy: Used for numerical operations, it helps with array operations that are crucial for backtesting algorithms.
- Matplotlib/Plotly: These libraries allow you to visualize data and strategy performance.
- ccxt: A popular library for interfacing with various cryptocurrency exchanges to fetch real-time and historical market data.
- Backtrader: A comprehensive backtesting library that integrates with various data sources, helping you simulate your strategies.
- TA-Lib: Provides technical analysis indicators like RSI, moving averages, and more, which are often integral to crypto trading strategies.
Installing the Environment
- Install Python 3.9 or later from the official Python website.
- Set up a virtual environment using the following command:
python -m venv crypto_env
- Activate the environment:
source crypto_env/bin/activate (Linux/macOS)
crypto_env\Scripts\activate (Windows)
- Install the required libraries via pip:
pip install pandas numpy matplotlib plotly ccxt backtrader ta-lib
- Ensure that all libraries are properly installed by checking their versions:
pip show pandas numpy matplotlib ccxt backtrader ta-lib
Important Considerations
Make sure your system can handle large datasets, especially when dealing with high-frequency data. If you're using real-time data feeds, you may need a more robust setup for handling asynchronous data fetching.
Table of Recommended Tools
Library | Purpose |
---|---|
Pandas | Data manipulation and analysis |
NumPy | Numerical operations for backtesting |
ccxt | Crypto exchange API integration |
Backtrader | Backtesting framework |
TA-Lib | Technical analysis indicators |
Integrating Cryptocurrency Data APIs for Real-Time Backtesting
For efficient real-time backtesting of cryptocurrency trading strategies, integrating external data sources is crucial. APIs (Application Programming Interfaces) are commonly used to access the raw data from cryptocurrency exchanges. These APIs provide up-to-date market data such as historical prices, order book depth, and trade volume, allowing developers to create robust and dynamic backtesting frameworks. Connecting to an API for real-time data is key to simulating live trading environments, ensuring the strategy’s performance is tested under realistic conditions.
Choosing the right API for integrating cryptocurrency data can significantly impact the accuracy and speed of backtesting. Many exchanges offer their own APIs, while third-party services provide aggregated data from multiple platforms. Understanding the data structure, rate limits, and latency is essential to optimize the backtesting process. By configuring APIs properly, developers can retrieve and process data in real-time to simulate trading scenarios and adjust their algorithms accordingly.
Key Considerations for API Integration
- Data Frequency: Ensure the API provides data with sufficient granularity (e.g., minute-level or tick-by-tick data) for realistic backtesting.
- Latency: Choose low-latency APIs for real-time strategy evaluation.
- Reliability: Consider APIs with high uptime to avoid interruptions during backtesting.
Steps for API Integration
- Choose the Right API: Select an API that supports the necessary data for your strategy, such as OHLC (Open, High, Low, Close) data, trade history, or order book snapshots.
- Set Up Authentication: Implement API keys or OAuth protocols for secure access.
- Handle Data Retrieval: Fetch and preprocess data at regular intervals to keep your backtesting environment in sync with real-time market movements.
- Manage Rate Limits: Ensure that the API is not overwhelmed by excessive requests by respecting rate limits.
Note: Always check the API documentation for any limits or restrictions to avoid unnecessary delays or failures during data retrieval.
Example API Data Structure
Field | Description |
---|---|
timestamp | The time of the market data in UTC. |
open | Opening price for the time period. |
high | Highest price during the time period. |
low | Lowest price during the time period. |
close | Closing price for the time period. |
volume | Trading volume for the time period. |
Designing Custom Crypto Trading Algorithms in Python
Creating efficient and profitable cryptocurrency trading strategies requires not only a deep understanding of the market, but also the ability to implement and backtest those strategies effectively. Python has become the go-to programming language for developing custom crypto trading algorithms due to its simplicity, extensive libraries, and robust ecosystem. In this context, designing algorithms with Python often involves using libraries such as Pandas, NumPy, and TA-Lib, alongside advanced techniques like machine learning for predictive modeling.
The process of building a custom crypto trading algorithm in Python typically includes several key steps: data collection, strategy development, backtesting, and optimization. This structured approach ensures that the algorithm performs well under various market conditions, and helps minimize the risk of financial loss during live trading.
Steps to Create a Custom Trading Algorithm
- Data Collection: Gather historical price data and relevant market indicators. This data forms the basis for strategy development and backtesting.
- Strategy Development: Design a trading strategy, which can be based on technical analysis, machine learning, or a combination of both.
- Backtesting: Test the strategy using historical data to evaluate its performance under different market conditions.
- Optimization: Fine-tune parameters to improve profitability while managing risk.
- Live Testing: Once optimized, test the algorithm in a live environment with minimal capital to monitor real-time performance.
Tools for Building Crypto Trading Algorithms
Library/Tool | Purpose |
---|---|
Pandas | Data manipulation and analysis |
NumPy | Numerical operations and mathematical functions |
TA-Lib | Technical analysis indicators |
ccxt | Connects to various crypto exchanges for live trading |
Scikit-learn | Machine learning algorithms for predictive modeling |
Important Tip: Always perform rigorous backtesting before deploying your strategy in a live environment to ensure it performs well under different market scenarios and is not overfitted to historical data.
Optimizing Backtest Speed: Python vs C++ for Crypto Strategies
When it comes to optimizing the performance of backtesting frameworks for cryptocurrency trading strategies, the speed of execution is a critical factor. Python, known for its simplicity and readability, has gained popularity in the field of quantitative finance. However, for strategies that demand high-speed calculations or handle vast amounts of data, C++ offers significant performance advantages due to its lower-level control over system resources.
Choosing between Python and C++ often depends on the complexity of the strategy and the scale of backtesting required. Python is suitable for rapid prototyping and testing smaller datasets, while C++ is more effective for large-scale backtesting, where execution speed becomes a bottleneck. Both languages have their strengths, but understanding their key differences is essential for making an informed decision about which to use for optimizing backtest speed.
Performance Comparison
Factor | Python | C++ |
---|---|---|
Execution Speed | Slower, interpreted language | Faster, compiled language |
Memory Efficiency | Less efficient, higher memory consumption | More efficient, lower memory overhead |
Ease of Development | Faster development, easier debugging | Slower development, more complex debugging |
Data Handling | Good for small to medium-sized datasets | Excellent for large-scale datasets |
Key Considerations
- Data Handling: Python excels in ease of use when handling small to medium data, thanks to libraries like Pandas. However, when datasets grow into millions of records, C++ can offer a significant speed advantage due to its optimized memory management and faster execution of heavy computations.
- Execution Time: Backtests with complex strategies and large datasets can be very slow in Python. C++ allows for finer control of computational resources, making it more suitable for high-frequency backtesting.
- Development Speed: While Python is easier to develop in and allows for rapid iterations, C++ can be more time-consuming due to the need to manually manage memory and optimize code.
For strategies that require intensive calculations, such as those utilizing machine learning or deep learning models, C++ provides the necessary speed to handle real-time backtesting at scale. Python, while slower, still remains the go-to choice for strategy research and initial testing.
Handling Market Data: Time Series & Price Feeds in Backtests
When building and testing cryptocurrency trading strategies, accurate and reliable market data is essential for simulating realistic trading conditions. In backtesting, the quality of time series data and price feeds plays a critical role in ensuring that the strategy behaves as expected under real market conditions. A well-constructed backtest relies on clean, high-frequency data to capture market movements, volatility, and liquidity changes over time. This is especially important when using Python or C++ for backtesting, as both languages provide tools to handle large datasets efficiently.
Market data for backtesting typically consists of time series, which represent the historical price movements of assets over time. These time series can be derived from different price feeds, such as bid/ask data, OHLC (Open, High, Low, Close), or tick-by-tick data. The choice of data granularity and frequency directly affects the backtest accuracy and performance, especially for high-frequency trading strategies. Below, we discuss the types of market data and their handling in backtests.
Types of Market Data
- OHLC Data: This data includes the open, high, low, and close prices for a given time period. It is commonly used in backtests for strategies that rely on candlestick charts.
- Tick Data: Contains every trade and price change, providing the most granular view of market movements. It is used for strategies that demand high precision.
- Bid/Ask Data: Records the best available bid and ask prices at each moment. This is crucial for simulating realistic order execution and slippage.
Considerations for Accurate Backtesting
- Data Integrity: Ensure that the data is free of errors, such as missing values, price jumps, or inaccuracies. Data cleansing methods are critical for reliable backtesting results.
- Data Frequency: Choose the right granularity of data. Higher-frequency data can capture more intricate market movements, while lower-frequency data may miss out on critical information.
- Market Hours: Ensure that the market data corresponds to the exact trading hours of the asset, especially for cryptocurrencies that trade 24/7.
Data Management in Python & C++
In both Python and C++, handling time series data for backtesting requires efficient storage and processing mechanisms. Common tools in Python include Pandas for time series manipulation and NumPy for numerical computations. These libraries allow for easy indexing and slicing of time series data to match the backtesting strategy requirements. In C++, developers often leverage libraries such as Boost for time series management and custom data structures to handle large-scale datasets.
Important: Always synchronize data when combining multiple sources, as discrepancies in time stamps can cause significant issues in the backtest, leading to misleading results.
Example of Market Data Structure
Time | Open | High | Low | Close | Volume |
---|---|---|---|---|---|
2025-04-17 00:00 | 46000 | 46500 | 45500 | 46050 | 1200 BTC |
2025-04-17 01:00 | 46050 | 46800 | 45900 | 46300 | 1150 BTC |
Debugging and Troubleshooting Backtesting Results in Python
When conducting backtesting of cryptocurrency trading strategies in Python, it's common to encounter issues that affect the accuracy of results. These issues can range from incorrect calculations to unexpected behavior in the code. Identifying and resolving these problems is critical to ensuring that the strategy performs as expected under historical market conditions. The debugging process often involves verifying data integrity, examining algorithm logic, and ensuring that the backtest execution environment is set up correctly.
Effective debugging starts with breaking down the backtesting workflow. A thorough inspection of key components, including data handling, order execution, and performance metrics, will help locate potential sources of discrepancies. Below are several key steps to help identify common issues when troubleshooting backtesting results.
Key Debugging Strategies
- Check Data Quality: Ensure that historical market data is accurate, complete, and free from gaps or anomalies. Missing data or incorrect timeframes can lead to erroneous backtest results.
- Review Algorithm Logic: Validate that the strategy's buy and sell conditions are correctly implemented. Mistakes in decision-making logic, such as incorrect threshold values or logical errors in trading signals, can affect performance.
- Examine Performance Metrics: Calculate and interpret various performance metrics such as Sharpe ratio, maximum drawdown, and total return. Discrepancies in these numbers may signal errors in the backtesting code.
Step-by-Step Debugging Process
- Start by isolating the issue: Are the results consistently incorrect, or does the problem occur intermittently?
- Log intermediate outputs: Print out key variables like entry/exit prices, portfolio value, and trade execution times to spot inconsistencies.
- Compare the backtest results with a manual calculation or a trusted third-party source to ensure accuracy.
- Refactor and test smaller code segments incrementally to verify that each component works correctly in isolation.
Common Pitfalls
Issues such as time-zone mismatches, incorrect order execution logic, or unaccounted slippage can lead to significant discrepancies in backtesting results. Always ensure that your backtest simulates real trading conditions as accurately as possible.
Sample Data Discrepancies
Issue | Potential Cause | Resolution |
---|---|---|
Missing price data | Incomplete data feed | Fill in missing values with interpolation or drop the affected periods |
Slippage not accounted for | Failure to simulate real-world trading costs | Include slippage in order execution logic |
Overfitting | Excessive parameter optimization | Test strategy robustness with out-of-sample data |
Leveraging C++ for High-Speed Crypto Strategy Backtesting
When developing sophisticated cryptocurrency trading strategies, time is often a critical factor. To evaluate the effectiveness of a strategy, traders must run simulations against vast amounts of historical data, which can be computationally expensive. By leveraging C++, developers can significantly reduce the time needed for backtesting, enabling more rapid iterations and optimizations. C++ excels in scenarios where performance and speed are paramount due to its low-level memory control and optimization capabilities.
Unlike Python, which is often favored for its ease of use and extensive libraries, C++ provides faster execution, particularly for computationally intensive tasks such as complex simulations of crypto trading strategies. This can be particularly beneficial for high-frequency or algorithmic trading systems, where every millisecond counts. Additionally, C++'s ability to integrate with Python allows for the use of both languages in a hybrid approach, combining Python's flexibility with C++'s performance.
Key Benefits of Using C++ in Crypto Strategy Simulations
- Speed: C++ provides near-instantaneous execution times, which is essential for backtesting large datasets quickly.
- Resource Efficiency: With direct memory management, C++ reduces overhead and optimizes CPU usage.
- Parallel Processing: C++ can utilize multi-threading and parallel computing for even faster simulation times.
- Precision: C++ allows for highly accurate floating-point calculations, essential for simulating complex strategies in the crypto market.
How C++ Improves Backtesting Performance
Backtesting cryptocurrency strategies involves processing large datasets, often containing millions of trades. C++ accelerates this process by offering more control over memory allocation and CPU cycles, enabling optimized algorithms for processing data in parallel.
"The main advantage of using C++ is the ability to fine-tune performance for high-frequency data, which is crucial for simulating strategies that require fast decision-making based on real-time market data."
For example, when simulating a trading algorithm that evaluates thousands of trades per second, using C++ results in dramatically reduced simulation times, compared to Python-based approaches. This translates to faster feedback loops and quicker adjustments to trading strategies, giving traders an edge in volatile crypto markets.
Example Comparison of Backtest Time Efficiency
Task | Python (seconds) | C++ (seconds) |
---|---|---|
Simulate 1 Million Trades | 600 | 60 |
Complex Risk Adjustment | 250 | 25 |
Real-time Strategy Simulation | 500 | 50 |
In this example, C++ is an order of magnitude faster than Python, making it an ideal choice for intensive simulations where time-to-result is critical for effective decision-making in crypto trading.